mirror of https://github.com/coder/coder.git
fix: Use /projects as the landing page (#72)
Previously, there was a pseudo-workspaces page that was leftover from prototyping, but doesn't make sense in the revised flow. Now, we have a `/projects` page, and after logging in, the user should be taken to that page: 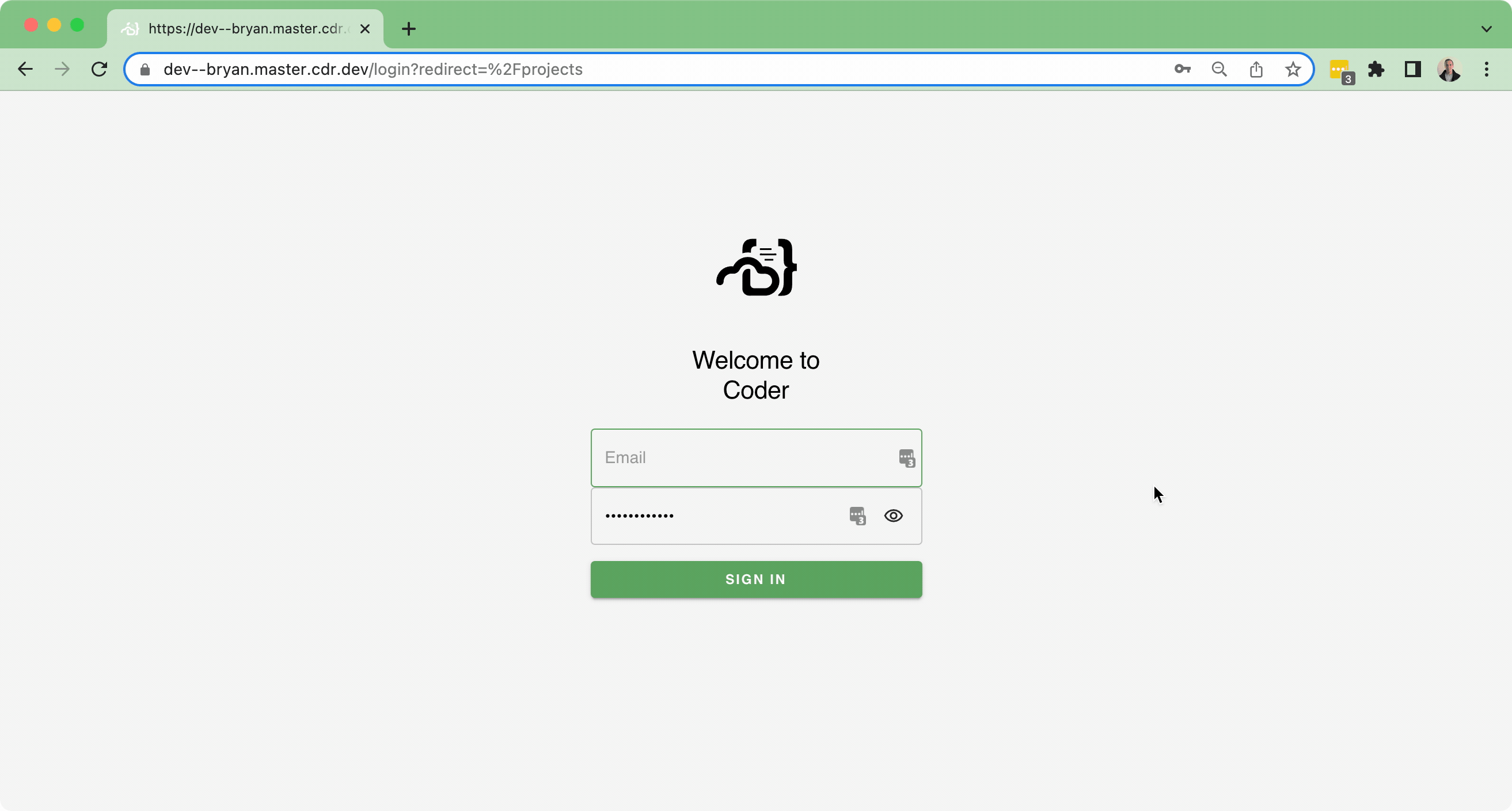 This implements a client-side redirect to land on our `/projects` route.
This commit is contained in:
parent
3047f251a8
commit
0c46cbf406
|
@ -0,0 +1,22 @@
|
|||
import { render, waitFor } from "@testing-library/react"
|
||||
import singletonRouter from "next/router"
|
||||
import mockRouter from "next-router-mock"
|
||||
import React from "react"
|
||||
import { Redirect } from "./Redirect"
|
||||
|
||||
describe("Redirect", () => {
|
||||
// Reset the router to '/' before every test
|
||||
beforeEach(() => {
|
||||
mockRouter.setCurrentUrl("/")
|
||||
})
|
||||
|
||||
it("performs client-side redirect on render", async () => {
|
||||
// When
|
||||
render(<Redirect to="/workspaces/v2" />)
|
||||
|
||||
// Then
|
||||
await waitFor(() => {
|
||||
expect(singletonRouter).toMatchObject({ asPath: "/workspaces/v2" })
|
||||
})
|
||||
})
|
||||
})
|
|
@ -0,0 +1,23 @@
|
|||
import React, { useEffect } from "react"
|
||||
import { useRouter } from "next/router"
|
||||
|
||||
export interface RedirectProps {
|
||||
/**
|
||||
* The path to redirect to
|
||||
* @example '/projects'
|
||||
*/
|
||||
to: string
|
||||
}
|
||||
|
||||
/**
|
||||
* Helper component to perform a client-side redirect
|
||||
*/
|
||||
export const Redirect: React.FC<RedirectProps> = ({ to }) => {
|
||||
const router = useRouter()
|
||||
|
||||
useEffect(() => {
|
||||
void router.replace(to)
|
||||
}, [])
|
||||
|
||||
return null
|
||||
}
|
|
@ -1,3 +1,4 @@
|
|||
export * from "./Button"
|
||||
export * from "./EmptyState"
|
||||
export * from "./Page"
|
||||
export * from "./Redirect"
|
||||
|
|
|
@ -1,78 +1,18 @@
|
|||
import React from "react"
|
||||
import Box from "@material-ui/core/Box"
|
||||
import { makeStyles } from "@material-ui/core/styles"
|
||||
import Paper from "@material-ui/core/Paper"
|
||||
import AddWorkspaceIcon from "@material-ui/icons/AddToQueue"
|
||||
|
||||
import { EmptyState, SplitButton } from "../components"
|
||||
import { Navbar } from "../components/Navbar"
|
||||
import { Footer } from "../components/Page"
|
||||
import { useUser } from "../contexts/UserContext"
|
||||
import { Redirect } from "../components"
|
||||
import { FullScreenLoader } from "../components/Loader/FullScreenLoader"
|
||||
import { useUser } from "../contexts/UserContext"
|
||||
|
||||
const WorkspacesPage: React.FC = () => {
|
||||
const styles = useStyles()
|
||||
const { me, signOut } = useUser(true)
|
||||
const IndexPage: React.FC = () => {
|
||||
const { me } = useUser(/* redirectOnError */ true)
|
||||
|
||||
if (!me) {
|
||||
return <FullScreenLoader />
|
||||
if (me) {
|
||||
// Once the user is logged in, just redirect them to /projects as the landing page
|
||||
return <Redirect to="/projects" />
|
||||
}
|
||||
|
||||
const createWorkspace = () => {
|
||||
alert("create")
|
||||
}
|
||||
|
||||
const button = {
|
||||
children: "New Workspace",
|
||||
onClick: createWorkspace,
|
||||
}
|
||||
|
||||
return (
|
||||
<div className={styles.root}>
|
||||
<Navbar user={me} onSignOut={signOut} />
|
||||
<div className={styles.header}>
|
||||
<SplitButton<string>
|
||||
color="primary"
|
||||
onClick={createWorkspace}
|
||||
options={[
|
||||
{
|
||||
label: "New workspace",
|
||||
value: "custom",
|
||||
},
|
||||
{
|
||||
label: "New workspace from template",
|
||||
value: "template",
|
||||
},
|
||||
]}
|
||||
startIcon={<AddWorkspaceIcon />}
|
||||
textTransform="none"
|
||||
/>
|
||||
</div>
|
||||
|
||||
<Paper style={{ maxWidth: "1380px", margin: "1em auto", width: "100%" }}>
|
||||
<Box pt={4} pb={4}>
|
||||
<EmptyState message="No workspaces available." button={button} />
|
||||
</Box>
|
||||
</Paper>
|
||||
<Footer />
|
||||
</div>
|
||||
)
|
||||
return <FullScreenLoader />
|
||||
}
|
||||
|
||||
const useStyles = makeStyles((theme) => ({
|
||||
root: {
|
||||
display: "flex",
|
||||
flexDirection: "column",
|
||||
},
|
||||
header: {
|
||||
display: "flex",
|
||||
flexDirection: "row-reverse",
|
||||
justifyContent: "space-between",
|
||||
margin: "1em auto",
|
||||
maxWidth: "1380px",
|
||||
padding: theme.spacing(2, 6.25, 0),
|
||||
width: "100%",
|
||||
},
|
||||
}))
|
||||
|
||||
export default WorkspacesPage
|
||||
export default IndexPage
|
||||
|
|
Loading…
Reference in New Issue